How to Create a Slot Machine Using HTML, CSS, and JavaScript
Learn how to create a slot machine using HTML, CSS, and JavaScript. This step-by-step guide covers the basics of slot game HTML code, adding functionality, and enhancing your project with sound and animations.
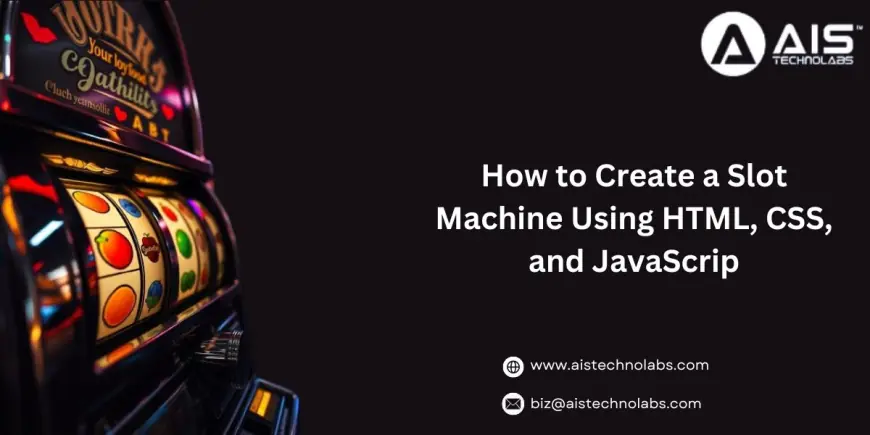
Slot machines are popular in gaming, and building one using web technologies like HTML, CSS, and JavaScript is a great way to learn coding. This guide will walk you through creating a functional slot machine using slot machine HTML code. By the end, you will have a basic slot game with spinning reels and winning logic.
1. Understanding the Basics of a Slot Machine
A slot machine consists of reels, symbols, and paylines. Players spin the reels, and matching symbols on paylines result in wins. Using slot game HTML code, you can recreate this experience in a browser. HTML structures the game, CSS styles it and JavaScript adds interactivity.
2. Setting Up the Project Structure
Start by creating a project folder. Inside, add three files: index.html, style.css, and script.js.
1. HTML File:
The HTML file defines the structure of the slot machine. Use <div> elements for reels and buttons for spinning.
2. CSS File:
The CSS file styles the reels, buttons, and layout. Use a flexbox or grid for alignment.
3. JavaScript File:
The JavaScript file handles the game logic, including spinning reels and checking wins.
3. Building the Slot Machine Interface
1. Designing the Reels:
Use <div> elements to represent each reel. Add symbols (like fruits or numbers) inside these elements.
2. Adding Buttons:
Create a "Spin" button to start the game and a "Reset" button to clear results.
3. Displaying Results:
Add a <div> to show messages like "You Win!" or "Try Again."
4. Implementing the Game Logic with JavaScript
1. Spinning the Reels:
Write a function to randomize symbols on each reel. Use Math.random() to generate random positions.
2. Checking Wins:
Define winning combinations (e.g., three matching symbols). Loop through the reels to check for matches.
3. Updating the Display:
Display win/lose messages and update the reels after each spin.
5. Enhancing the Slot Machine
1. Adding Sound Effects:
Use the <audio> tag to play sounds for spinning reels and wins.
2. Incorporating Animations:
Use CSS animations to make the reels spin smoothly.
3. Implementing a Points System:
Track points for wins and display them on the screen.
6. Testing and Debugging
1. Cross-Browser Testing:
Test the game in different browsers to ensure compatibility.
2. Debugging Issues:
Check for errors like reels not spinning or results not displaying.
3. Optimizing Performance:
Compress images and audio files to improve load times.
7. Making the Game Responsive
1. Mobile-Friendly Design:
Use media queries to adjust the layout for smaller screens.
2. Cross-Device Compatibility:
Test the game on smartphones, tablets, and desktops.
Conclusion
Creating a slot machine using HTML, CSS, and JavaScript is a fun way to learn web development. By following this guide, you can build a basic slot game with slot machine HTML code and enhance it with sound effects and animations. For more advanced features or custom projects, contact AIS Technolabs.
FAQ
1. What is a slot machine?
A slot machine is a game with reels and symbols. Players spin the reels to match symbols and win.
2. Can I build a slot machine without JavaScript?
No, JavaScript is essential for adding interactivity and game logic.
3. How do I add sound effects to my slot machine?
Use the <audio> tag in HTML and trigger sounds with JavaScript.
4. Is it hard to make a slot machine responsive?
No, use CSS media queries to adjust the layout for different screen sizes.
5. Can I add more features to my slot machine?
Yes, you can add animations, a points system, or bonus rounds.