Which One Should I Learn First: C or C++?
C and C++ are two of the most widely used programming languages. C, developed in the early 1970s, is known for its simplicity and efficiency.
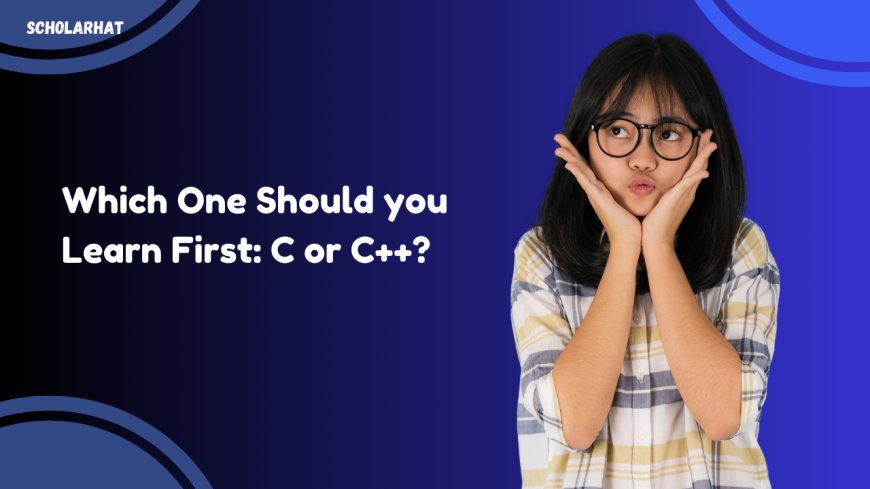
C and C++ are two of the most widely used programming languages. C, developed in the early 1970s, is known for its simplicity and efficiency. C++, developed as an extension of C in the 1980s, adds object-oriented features and other enhancements. Understanding their differences and unique features can help you choose the right starting point.
Overview of C
C is a procedural programming language that provides a solid foundation for understanding fundamental programming concepts. It is widely used in system programming, embedded systems, and other performance-critical applications.
Key Features of C:
-
Simple and straightforward syntax
-
Low-level memory access with pointers
-
Procedural programming paradigm
-
Efficient and fast execution
Overview of C++
C++ builds on C by adding object-oriented programming (OOP) features, making it a more versatile language. It supports both procedural and object-oriented programming, offering a broader range of programming paradigms.
Key Features of C++:
-
Supports OOP with classes and objects
-
Includes features from C (backward compatibility)
-
Standard Template Library (STL) for data structures and algorithms
-
Enhanced memory management and type safety
Key Differences Between C and C++
Feature |
C |
C++ |
Programming Paradigm |
Procedural |
Procedural and Object-Oriented |
Memory Management |
Manual with pointers |
Enhanced with constructors/destructors |
Standard Library |
Limited |
Extensive with STL |
Data Abstraction |
No native support |
Supports classes and objects |
Recursion |
Supported |
Supported |
Switch Statement |
Supported |
Supported |
Learning Curve and Complexity
Learning C
-
Pros:
-
Simpler syntax and fewer concepts to grasp.
-
Provides a strong foundation for understanding low-level programming.
-
Easier to learn for beginners with no programming experience.
-
Cons:
-
Lacks advanced features found in modern programming languages.
-
Requires manual memory management, which can lead to errors.
Learning C++
-
Pros:
-
Offers a mix of procedural and object-oriented programming.
-
Richer feature set with the Standard Template Library (STL).
-
Better for understanding modern programming paradigms.
-
Cons:
-
More complex syntax and steeper learning curve.
-
Object-oriented concepts can be challenging for beginners.
Use Cases and Applications
C
-
System Programming: Operating systems, embedded systems, and hardware drivers.
-
Performance-Critical Applications: High-performance computing tasks where efficiency is paramount.
-
Academic Use: Often taught as an introductory programming language in computer science courses.
C++
-
Game Development: Popular for developing game engines and real-time simulations.
-
Application Software: Widely used in developing desktop applications, like Adobe Photoshop.
-
High-Performance Software: Financial systems, real-time simulations, and complex data analysis.
Key Concepts in C
Recursion in C
Recursion is a technique where a function calls itself to solve smaller instances of a problem. It is widely used in C for tasks like traversing data structures and solving mathematical problems.
Example of Recursion in C:
#include
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int number = 5;
printf("Factorial of %d is %d\n", number, factorial(number));
return 0;
}
Switch Statement in C
The switch statement in C allows multi-way branching, making it easier to execute different code blocks based on the value of a variable.
Example of Switch Statement in C:
#include
int main() {
int choice = 2;
switch (choice) {
case 1:
printf("You chose option 1\n");
break;
case 2:
printf("You chose option 2\n");
break;
case 3:
printf("You chose option 3\n");
break;
default:
printf("Invalid choice\n");
}
return 0;
}
Conclusion
Choosing between C and C++ depends on your goals and interests. If you want to start with a simpler language that provides a strong foundation in programming concepts and low-level programming, C might be the right choice. However, if you are interested in learning modern programming paradigms and working on a wider range of applications, starting with C++ could be more beneficial.
Both languages offer valuable skills and knowledge, and learning either will provide a solid base for advancing to other programming languages and technologies. Ultimately, mastering both C and C++ will make you a versatile and well-rounded programmer.