How to Use Python and Node.js for Telegram Game Development
How to Use Python and Node.js for Telegram Game Development
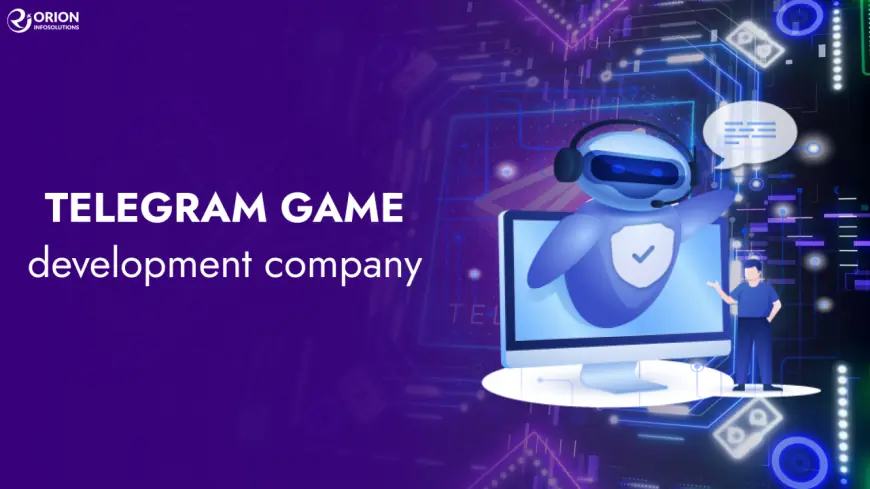
Telegram has emerged as a powerful platform for game development, allowing developers to create interactive bot-based games. As a Telegram game development company, choosing the right programming language is essential for building efficient, scalable, and engaging games. Two of the most popular languages for Telegram game development are Python and Node.js, both offering unique advantages in bot interaction, backend development, and real-time gameplay.
Why Use Python and Node.js for Telegram Game Development?
Both Python and Node.js provide robust tools and frameworks for creating Telegram-based games:
- Python: Best for text-based games, AI-powered interactions, and bot automation.
- Node.js: Ideal for real-time multiplayer games, asynchronous processing, and fast API interactions.
Let’s explore how to use Python and Node.js to build engaging Telegram games.
Building Telegram Games with Python
Python is one of the most widely used languages for Telegram bot and game development due to its simplicity and rich ecosystem.
Step 1: Setting Up Python and Installing Required Libraries
To start developing a Telegram game using Python, install the required libraries:
This library allows developers to interact with the Telegram Bot API.
Step 2: Creating a Telegram Bot
- Go to BotFather on Telegram.
- Use the
/newbot
command to create a bot and get an API token. - Save the token to authenticate your bot.
Step 3: Writing the Game Logic
Here’s a simple Python script to create a quiz-based game:
This script starts a simple question-answer game on Telegram.
Step 4: Deploying the Game
Deploy the bot on a cloud server like AWS, Heroku, or DigitalOcean to keep it running 24/7.
Building Telegram Games with Node.js
Node.js is a great choice for real-time, interactive Telegram games, thanks to its asynchronous nature.
Step 1: Setting Up Node.js and Installing Dependencies
First, install the required packages:
The node-telegram-bot-api package is used to interact with the Telegram API.
Step 2: Creating a Telegram Bot
- Get an API token from BotFather on Telegram.
- Use this token in your Node.js script.
Step 3: Writing the Game Logic
Here’s an example of a rock-paper-scissors game using Node.js:
This script allows users to play Rock-Paper-Scissors with the bot.
Step 4: Deploying the Game
Host the bot on a cloud platform like Heroku, AWS, or Vercel to ensure 24/7 availability.
Python vs. Node.js: Which One Should You Choose?
Feature | Python | Node.js |
---|---|---|
Ease of Use | ✅ Easy to learn | ???? Requires JavaScript knowledge |
Performance | ???? Slower than Node.js | ✅ Fast, handles real-time requests |
Best For | ✅ Text-based and AI-powered games | ✅ Real-time multiplayer games |
Libraries | ✅ Rich AI and bot libraries | ✅ Strong ecosystem for web apps |
Deployment | ???? Requires VPS or cloud | ✅ Easily deployable on cloud services |
- Use Python for text-based, AI-driven, or turn-based Telegram games.
- Use Node.js for real-time, fast-paced multiplayer games.
Conclusion
Both Python and Node.js offer powerful tools for Telegram game development. As a Telegram game development company, your choice depends on the type of game you want to build. Python is best suited for trivia, text-based, and AI-driven games, while Node.js is ideal for real-time interactive games. By leveraging these technologies effectively, you can create engaging and scalable Telegram games that captivate users and keep them coming back for more.