Java Testing Frameworks: An In-Depth Exploration of JUnit and TestNG
Explore the differences between JUnit and TestNG, two leading Java testing frameworks. Make informed choices for robust Java software testing.
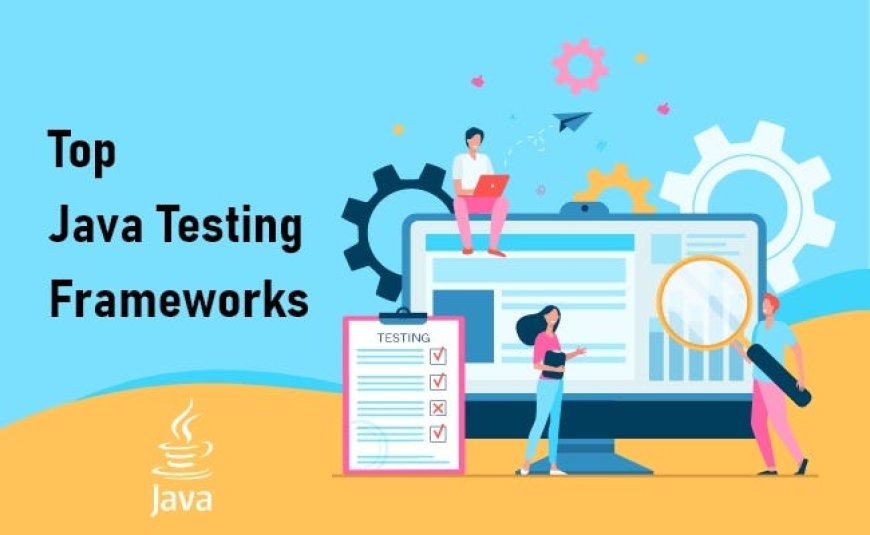
Introduction
Welcome to the fascinating world of Development, where crafting powerful and reliable software is both an art and a science. If you're just starting on this journey, you've likely encountered the term "testing frameworks." Don't let this technical jargon intimidate you – think of testing frameworks as your trustworthy companions on the quest for building robust and error-free Java applications.
Imagine you're embarking on a culinary adventure. Before serving a delightful dish, you meticulously check each ingredient's quality and taste. In the Java realm, testing frameworks, such as JUnit and TestNG, serve a similar purpose – ensuring the quality and functionality of your code.
JUnit, our first hero in this tale, is like the precision chef's knife in your kitchen. It specialises in unit testing, examining individual components of your code (we call them units) to make sure they perform as expected. Think of it as the chef tasting each ingredient separately before blending them into a harmonious dish.
J-Unit
JUnit is one of the most popular testing frameworks for Java, and it has been a cornerstone in the development of robust and maintainable code for many years. As a beginner, you might wonder why testing is necessary and what role JUnit plays in this process. Testing is an essential part of the software development life cycle, allowing developers to identify and fix bugs early in the process. JUnit, specifically designed for unit testing, enables developers to write and run tests on individual units or components of their code. A unit in this context refers to the smallest testable part of an application.
-
Annotations :
JUnit communicates through annotations, special labels that guide its actions. Annotations like @Test, @Before, and others act as your culinary instructions, detailing what needs to be tested and how to prepare for it.
@Test
public void testAddition() {
// Like a chef ensuring that adding ingredients results in the perfect flavour
}
-
Assertions :
JUnit introduces assertions, akin to the chef checking that the dish matches their culinary vision. These assertions, such as assertEquals, validate that the output aligns with expectations.
@Test
public void testAddition() {
int result = Calculator.add(2, 3);
assertEquals(5, result); // Ensuring the sum is as anticipated
}
-
Test Runners :
JUnit employs test runners to orchestrate the testing process. It's like having a seasoned sous-chef ensuring each step is executed in the right sequence.
@RunWith(Parameterized.class)
public class ParameterizedTest {
// Imagine testing a recipe with variations – this is your parameterized test
}
Now, let's shift our attention to TestNG, the sophisticated elder sibling of JUnit. While JUnit excels in unit testing, TestNG extends its capabilities to functional and end-to-end testing, making it a versatile tool for a broader spectrum of testing scenarios.
TestNG
As you set out to build applications, envision TestNG as your guiding compass, leading you through uncharted territories of testing with finesse and flexibility. Testing frameworks, in essence, are the vigilant guardians of your code's integrity, ensuring it stands strong against the ever-changing tides of development.
TestNG, a testing framework that extends beyond conventional boundaries, offering an array of features designed to meet the dynamic demands of modern software development.
-
Annotations :
TestNG shares the concept of annotations with JUnit, but it takes this idea to the next level. Annotations in TestNG are not merely markers; they are powerful directives that allow you to fine-tune your tests.
@Test Annotation :
@Test
public void testMultiplication() {
// Your test logic goes here }
Similar to JUnit's @Test, this annotation signals that the method is a test case. However, TestNG adds more granularity and flexibility to this annotation.
-
@BeforeTest and @AfterTest :
@BeforeTest
public void setUp() {
// Actions performed before the test suite runs
}
@AfterTest
public void cleanUp() {
// Actions performed after the test suite runs
}
These annotations let you set up and clean up resources at the beginning and end of the entire test suite, providing a broader scope than JUnit's @BeforeClass and @AfterClass.
-
@BeforeMethod and @AfterMethod :
@BeforeMethod
public void beforeEachTest() {
// Actions performed before each test method
}
@AfterMethod
public void afterEachTest() {
// Actions performed after each test method
}
These annotations allow you to define preconditions and cleanup steps specifically for each test method.
-
Parameterized Testing: Testing with Variety
TestNG simplifies parameterized testing, making it more accessible and intuitive.
@Test(dataProvider = "testData")
public void testParameterized(int input1, int input2, int expected) {
// Your parameterized test logic goes here
}
@DataProvider(name = "testData")
public Object[][] provideTestData() {
// Your data provider logic goes here
}
In this example, the @DataProvider annotation is used to supply test data to the parameterized test. This feature allows you to run the same test with different inputs seamlessly.
"Enrol Now “Java course in Pune” to learn about JUnit and Test-NG"
JUnit vs Test-NG
As a beginner in Java development, understanding the nuances of testing frameworks is pivotal. Two prominent players in this arena are JUnit and TestNG. Let's embark on a comparative journey to explore their strengths, use cases, and distinctive features.
-
JUnit: Focus: Primarily designed for unit testing, examining individual units or components of code.
Philosophy: Emphasises simplicity and ease of use for testing individual methods and classes.
-
TestNG: Focus: Encompasses unit, functional, and end-to-end testing, providing a broader scope than JUnit.
Philosophy: Aims to be a comprehensive testing framework with additional features for various testing scenarios.
-
JUnit: Annotations: Uses annotations such as @Test, @Before, @After, @BeforeClass, and @AfterClass for configuration. Granularity: Annotations provide basic configuration but are less granular compared to TestNG.
-
TestNG: Annotations: Offers a rich set of annotations including @Test, @BeforeTest, @AfterTest, @BeforeMethod, and @AfterMethod. Granularity: Annotations provide fine-grained control over test configuration, making it more flexible.
-
JUnit: Parameterized Tests: Requires the use of external libraries like Parameterized to enable parameterized testing. Complexity: Parameterized tests can be more complex to set up.
-
TestNG: Parameterized Tests: Built-in support for parameterized testing, simplifying the process. Ease of Use: Offers a straightforward way to run the same test with different inputs.
-
JUnit: Grouping: Does not have native support for test groups. Workaround: Achieved through naming conventions or external tools.
-
TestNG: Grouping: Introduces the concept of test groups, allowing easy categorization of tests. Flexibility: Enables selective execution of tests based on groups, enhancing test organisation.
-
JUnit: Parallel Execution: Requires external tools or frameworks for parallel test execution. Complexity: Setting up parallel execution can be more involved.
-
TestNG: Parallel Execution: Native support for parallel test execution. Configuration: Offers straightforward configuration for parallel execution, reducing test time.
Conclusion
JUnit is an excellent choice for beginners and projects primarily focused on unit testing. Its simplicity makes it easy to pick up and use for straightforward testing scenarios.
TestNG, on the other hand, shines when your testing requirements extend beyond unit testing. If you anticipate the need for parameterized tests, test grouping, parallel execution, and enhanced reporting, TestNG provides a more comprehensive toolset.
Ultimately, the choice between JUnit and TestNG depends on the specific needs and complexities of your Java project. As you gain experience, experimenting with both frameworks will deepen your understanding and equip you to make informed decisions based on the unique demands of your software development endeavours. Happy testing!