How to Create Simple Calculator using Javascript HTML, CSS
Learn how to build a simple calculator using JavaScript! This beginner-friendly guide covers everything you need to know, including basic arithmetic operations, DOM manipulation, event handling, and reusable functions. Start your JavaScript journey with this hands-on project!
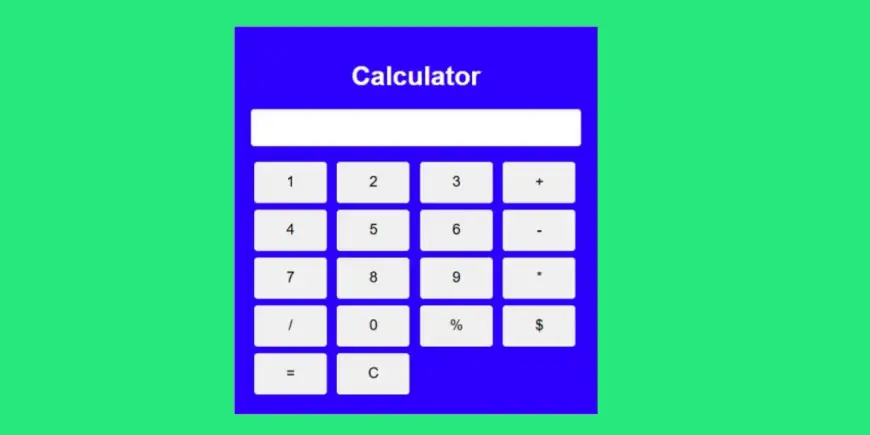
JavaScript is one of the most versatile programming languages, widely used for creating dynamic and interactive web applications. If you're a beginner looking to strengthen your understanding of JavaScript, creating a simple calculator using JavaScript is a fantastic project to start with. This tutorial will guide you through building a functional calculator with a sleek interface while providing insights into the JavaScript concepts involved.
Why Build a Calculator?
A calculator may seem like a basic project, but it covers fundamental concepts of programming, such as:
- Handling user inputs.
- Performing basic arithmetic operations.
- Manipulating the DOM (Document Object Model).
- Understanding events and event listeners.
- Writing clean, reusable functions.
Once you’ve completed this project, you’ll have a better grasp of how JavaScript interacts with HTML and CSS to create dynamic web applications.
The Anatomy of a Simple Calculator
A simple calculator typically includes:
- Buttons for Digits and Operators: Number keys (0-9) and basic arithmetic operators like addition (+), subtraction (-), multiplication (*), and division (/).
- Display Screen: A screen or area to display the input and results.
- Functional Buttons: A button for clearing the display and another to compute the result (e.g., an "=" button).
Before diving into JavaScript, you’ll need to set up the calculator's structure using HTML and style it with CSS. However, our focus here will be on how JavaScript makes the calculator functional.
Adding JavaScript for Functionality
The real magic happens in JavaScript. Let’s break down the essential steps:
- Getting References to DOM Elements: Use
document.querySelector
ordocument.getElementById
to access the display area and buttons. - Adding Event Listeners: Listen for button clicks and determine the type of button pressed (digit, operator, or special function).
- Handling Input: Update the display with the button value when a digit or operator is pressed.
- Performing Calculations: When the "=" button is clicked, evaluate the expression in the display using the
eval()
function. - Clearing the Display: Reset the calculator when the "C" button is clicked.
Here’s an outline of the core logic:
- Storing Inputs: Maintain a variable to track user inputs and update it whenever a button is clicked.
- Evaluating Expressions: Use
eval()
to compute the result of the entered expression. - Error Handling: Handle invalid expressions gracefully by displaying an error message.
Best Practices When Using JavaScript
- Avoid Using eval() for Production: Although
eval()
simplifies evaluating expressions, it poses security risks. For production-grade projects, consider using safer alternatives like implementing a custom parser. - Organize Code for Readability: Divide your JavaScript code into functions for clarity and reusability. For instance, create separate functions for handling input, updating the display, and clearing the screen.
- Validate User Input: Ensure the user cannot enter invalid combinations of operators (e.g.,
++
or/*
) by validating the input before updating the display.
Additional Features to Enhance the Calculator
Once you’ve built a basic calculator, consider adding more features to make it robust:
- Advanced Functions: Add buttons for square roots, percentages, and power calculations.
- Keyboard Support: Allow users to operate the calculator using their keyboard, improving accessibility.
- Theming: Use CSS to implement light and dark modes for a modern user experience.
- Error-Free Input Handling: Implement logic to prevent consecutive operators and ensure proper use of decimals.
Testing Your Calculator
Testing is crucial to ensure your calculator works seamlessly. Here are some scenarios to test:
- Basic arithmetic operations (e.g.,
2 + 2
,10 / 2
). - Clearing the display and starting over.
- Chaining multiple operations (e.g.,
5 + 3 - 2
). - Handling invalid input gracefully (e.g.,
/ 5
without a preceding number).
Conclusion
Moreover, this project lays a strong foundation for more complex applications, making it an ideal starting point for beginners.
Once your calculator is functional, don’t stop there! Experiment with additional features and styling to challenge yourself further. By continually iterating on your project, you’ll gain confidence and proficiency in JavaScript and web development as a whole.
Watch video on youtube : Javascript Simple Calculator