Dockerizing Laravel: A Step-by-Step Guide to Containerization
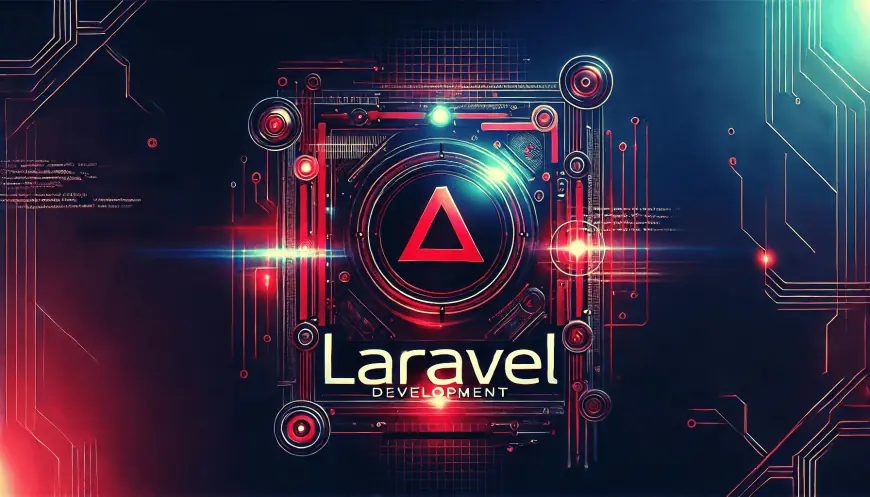
Hey folks! If you're working with Laravel and want a smoother development experience, Docker is your best friend. In this guide, we'll walk through containerizing a Laravel application, making deployment seamless and efficient. Whether you're a solo developer or part of a Laravel development company, containerization will help you eliminate "it works on my machine" problems once and for all. Let's dive in!
Why Docker is Essential for Laravel Development
Docker isn't just a buzzword—it's a game-changer for Laravel development. It provides a consistent environment across different systems, ensuring that your application runs the same way on every machine, from local development to production.
Simplified Laravel Development
Imagine setting up a Laravel project without worrying about mismatched PHP versions, conflicting dependencies, or different OS configurations. Docker packages everything—Laravel, PHP, MySQL/PostgreSQL, Redis—into isolated containers, making onboarding new developers a breeze.
Key Benefits of Using Docker for Laravel
-
Scalability: Spin up multiple instances effortlessly to handle traffic spikes.
-
Dependency Management: No more "works on my machine" issues—every developer gets the same setup.
-
Version Control: Define and lock specific PHP, database, and package versions to avoid unexpected updates breaking your app.
-
Faster Deployment: Reduce setup time and deploy your Laravel applications faster with pre-configured environments.
How Docker Works with Laravel
Docker works by creating lightweight containers that run isolated applications. Instead of installing Laravel and its dependencies directly on your system, Docker uses images to define an environment, ensuring consistency across machines.
Containers, Images, and Docker Compose
-
Docker Images: Blueprints for creating containers (like a Laravel image with PHP and Nginx pre-installed).
-
Docker Containers: Running instances of images, each functioning like a mini virtual machine.
-
Docker Compose: A tool for managing multi-container applications (Laravel, MySQL, Redis, etc.) with a single YAML configuration file.
Why Laravel Developers Should Use Docker
Developing Laravel applications in Docker eliminates local environment conflicts. You can switch PHP versions, use Redis, or test with different database engines without modifying your system setup. Plus, Docker makes deployment seamless across dev, staging, and production environments.
Key Components of a Dockerized Laravel Application
A Laravel application inside Docker typically consists of the following components:
Component |
Role in Laravel Docker Environment |
Nginx |
Web server handling requests efficiently. |
PHP-FPM |
Fast processing of PHP scripts, improving performance. |
MySQL/PostgreSQL |
Primary database storing Laravel application data. |
Redis |
Caching and queue management for faster performance. |
Each of these plays a crucial role in optimizing Laravel performance, ensuring that your app runs smoothly in production.
Setting Up Docker for Laravel
Before you start, ensure you have Docker and Docker Compose installed. You can download them from Docker's official site.
Installing Docker & Docker Compose
Windows/macOS: Download and install Docker Desktop.
Linux: Install using:
sh |
Creating a Docker Environment for Laravel
Once Docker is installed, create a dockerized Laravel project by initializing a Dockerfile and docker-compose.yml.
Writing a Dockerfile for Laravel
A Dockerfile is a blueprint for building a Docker image. It defines the Laravel environment and required dependencies.
Purpose of a Dockerfile
The Dockerfile specifies:
-
Base OS (e.g., Alpine or Ubuntu)
-
PHP version and extensions
-
Composer installation
-
Laravel dependencies
Example Dockerfile
Dockerfile |
Configuring Docker Compose for Laravel
Docker Compose simplifies multi-container setups, allowing you to define Laravel, MySQL, Redis, and other services in a single YAML file.
Example docker-compose.yml
yaml |
Running Laravel in Docker
Now, let's get our Laravel application running inside a Docker container.
Building and Running the Laravel Container
Run the following command to start the services:
sh |
This will spin up your Laravel application along with its database and dependencies.
Connecting Laravel to Services
Update your .env file to ensure Laravel connects to the correct database container:
env |
Now, run migrations:
sh |
Managing Laravel Queues and Background Jobs in Docker
Laravel queues improve performance by handling long-running tasks in the background.
Running Laravel Queues with Supervisor
Inside the Docker container, use Supervisor to manage queue workers:
sh |
Then, create a Supervisor config:
ini |
Restart Supervisor:
sh |
Final Thoughts
Dockerizing Laravel simplifies development, deployment, and scaling. With consistent environments, automated dependency management, and seamless service integration, Laravel in Docker is a must for modern web applications. Whether you're a freelancer or part of a Laravel web development services team, embracing Docker will boost efficiency.
Key Takeaways
-
Docker eliminates environment-related issues in Laravel development.
-
Essential components include PHP-FPM, Nginx, MySQL/PostgreSQL, and Redis.
-
Docker Compose makes managing multiple services easier.
Frequently Asked Questions (FAQs)
1. Why should I use Docker for Laravel?
Docker ensures that your Laravel application runs identically across different environments. It eliminates dependency conflicts, simplifies local development, and makes deployment seamless, whether for a small project or enterprise-level application.
2. How do I set up Docker for Laravel development?
Install Docker and Docker Compose, then create a Dockerfile and docker-compose.yml file. Define your Laravel app’s dependencies and run docker-compose up -d to start your containerized environment.
3. What is the best Docker Compose setup for Laravel?
The ideal Docker Compose setup includes services for PHP, MySQL/PostgreSQL, Redis, and Nginx. This ensures optimized performance, efficient queue handling, and a seamless development workflow.
4. Can I use Docker for Laravel queues and cron jobs?
Yes! You can run Laravel queues inside a Docker container using Supervisor. Define a process to monitor and restart queue workers, ensuring background jobs execute smoothly without manual intervention.
5. How does Docker improve Laravel performance?
Docker enhances Laravel performance by optimizing resource usage, isolating dependencies, and ensuring faster deployments. It also improves scalability by making it easier to spin up multiple instances when needed.
Now that you know how to Dockerize your Laravel application, why not give it a shot? Happy coding!