The Ultimate Guide to Best Design Patterns in React for 2025
Discover the top React design patterns for 2025 to boost performance, scalability and maintainability in your web development projects.
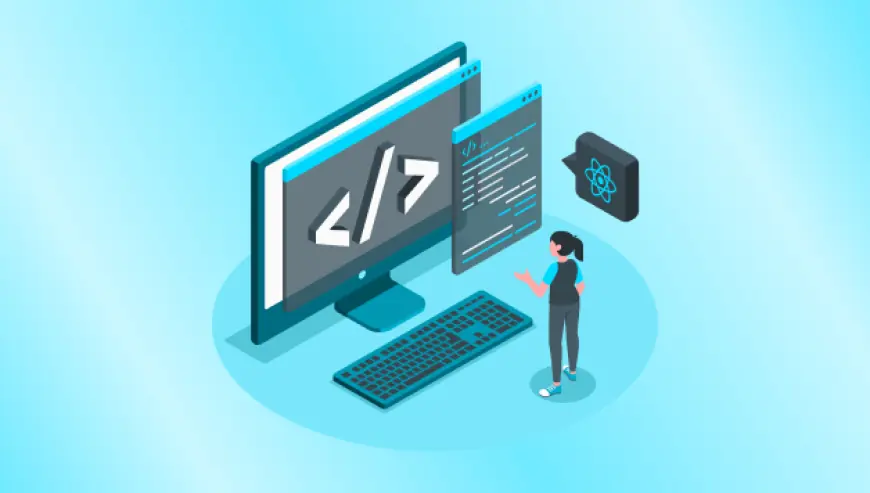
Mastering React design patterns remains an important skill for every developer in today's growing world of web development because the more popular JavaScript library nowadays is definitely React. Hence, through knowledge and proper implementation, it ensures code maintainability and scalability and also has better app performance. In this comprehensive guide, we will go through the core concepts of React design patterns, discuss best practices, and provide insights that will help you build better React applications in 2025.
What Are React Design Patterns?
React design patterns are tried-and-tested solutions to common problems encountered during React application development. These patterns act as reusable templates that streamline the coding process and make your application more organized. By leveraging these patterns, developers can write cleaner, more efficient code while adhering to industry standards.
Why Are Design Patterns in React Important?
As applications become increasingly complex, keeping a consistent structure is difficult. In React, design patterns guide the proper arrangement of code, reduce repetition, and enhance the readability of code. They make it easier for teams to:
-
Streamline Development: Standardized approaches make the work easy for developers in collaboration.
-
Reduce Errors: Predefined solutions minimize bugs.
-
Improve Maintainability: Structured code makes it easy to update and debug.
-
Optimize Performance: Proper patterns make applications faster and more responsive.
Common React Design Pattern for 2025
Some popular React design pattern and best practices to include in your projects:
1. Component Composition Pattern
One of the core ideas behind React is breaking up the UI into small, composable components. Instead of constructing a huge monolithic component, you break it up into small, focused ones that you can compose together to form complex UIs.
const Button = ({ onClick, children }) => (
<button onClick={onClick}>{children}</button>
);
const App = () => (
<Button onClick={() => alert('Clicked!')}>Click Me</Button>
);
This pattern encourages more reusability and easier testing.
2. Higher-Order Components (HOCs)
HOCs are the advanced React design patterns to reuse the component logic. They accept a component as an argument and return a new component with extra functionality.
Example:
const withLogging = (WrappedComponent) => {
return (props) => {
console.log('Rendering', WrappedComponent.name);
return <WrappedComponent {.props} />;
};
};
const Hello = () => <div>Hello World!</div>;
const EnhancedHello = withLogging(Hello);
HOCs assist in managing issues such as logging, authentication, etc.
3. Render Props Pattern
The render props pattern is the sharing of code between components using a prop that is a function. This pattern is handy when you want to get dynamic behavior based on some conditions.
Example:
const MouseTracker = ({ render }) => {
const [position, setPosition] = React.useState({ x: 0, y: 0 });
const handleMouseMove = (event) => {
setPosition({ x: event.clientX, y: event.clientY });
};
return <div onMouseMove={handleMouseMove}>{render(position)}</div>;
};
const App = () => (
<MouseTracker render={({ x, y }) => (
<h1>The mouse position is ({x}, {y})</h1>
)} />
);
This pattern supports reusable and flexible components.
4. Context API Pattern
Context API is the effective way to manage global state in React applications. Instead of prop-drilling, you can use context to share data across components.
Example:
const ThemeContext = React.createContext('light');
const App = () => (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
const Toolbar = () => (
<ThemeContext.Consumer>
{(value) => <div>Current theme: {value}</div>}
</ThemeContext.Consumer>
);
Context API is a lean and mighty alternative to the third-party state management libraries.
5. Custom Hooks Pattern
Thanks to the hooks, new React design patterns have come into life. The custom hooks make it possible to extract the reusable logic from the component, hence making it cleaner and more manageable.
Here is the example:
const useCounter = () => {
const [count, setCount] = React.useState(0);
const increment = () => setCount(count + 1);
return { count, increment };
};
const Counter = () => {
const { count, increment } = useCounter();
return <button onClick={increment}>Count: {count}</button>;
};
This is a great pattern for abstracting repetitive logic.
React Design Patterns and Best Practices for 2025
To get the most from React design patterns, apply the following best practices:
-
Modularity: Components should have a single responsibility.
-
TypeScript: Static typing will reduce errors and increase productivity in developers.
-
Write Tests: Ensure your components and patterns work as intended.
-
Dry Principle: Refrain from duplicating the same logic across different components.
-
Performance Optimizations: Use tools like React Profiler for identifying and fixing bottlenecks.
At Glorywebs, we stress the need to implement modern React design patterns and best practices that ensure high-quality, scalable web solutions. With such approaches, your applications are sure to meet the stringent demands of 2025's fast-changing tech environment.
Conclusion
Design patterns are essential tools for React developers with the intention of building stable and maintainable applications. Understanding and applying these patterns can streamline development, improve code quality, and create user-friendly applications. As you work with design patterns in React, don't forget to continue learning and adapt to the latest trends. For further insight, visit our blog at React Design Patterns Explained, which talks about how Glorywebs can make your web development work more efficient.